Loading... ## 函数对象适配器 <div class="tip inlineBlock warning"> 函数适配器bind1st bind2nd 现在我有这个需求 在遍历容器的时候,我希望将容器中的值全部加上100之后显示出来,怎么做? 我们直接给函数对象绑定参数 编译阶段就会报错 for_each(v.begin(), v.end(), bind2nd(myprint(),100)); 如果我们想使用绑定适配器,需要我们自己的函数对象继承binary_function 或者 unary_function 根据我们函数对象是一元函数对象 还是二元函数对象 </div> ```C++ #include<iostream> #include<vector> #include<algorithm> #include<functional> using namespace std; //函数对象适配器 class MyPrint : public binary_function<int,int,void> { public: void operator()(int value,int start)const { cout << "value = " << value << " " << "start = " << start << " " << "sum = " << value + start << endl; } }; int main() { vector<int> v; int num; cout << "请输入起始值:" << endl; cin >> num; for (int i = 0; i < 20; i++) { v.push_back(i); } //1、需要将参数进行绑定 bind2nd for_each(v.begin(), v.end(), bind2nd(MyPrint(), num)); /*绑定顺序相反-<类型n,类型n-1,...,类型1>*/ //bind1st绑定是相反的 cout << "\n\n\n\n"; for_each(v.begin(), v.end(), bind1st(MyPrint(), num)); //2、将类进行一个继承 binary_function<类型1,类型2...,类型n,函数返回值> //3、加const return 0; } ``` 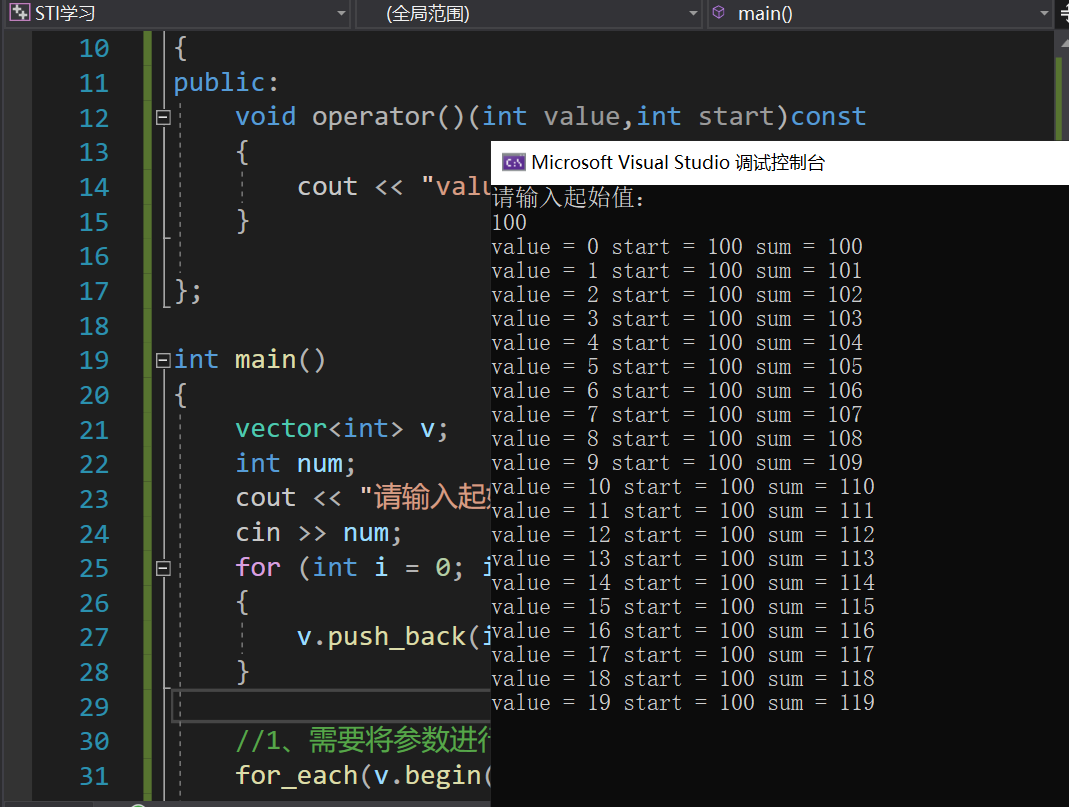 <div class="tip inlineBlock success"> 总结: bind1st和bind2nd区别? 1. bind1st : 将参数绑定为函数对象的第一个参数 2. bind2nd : 将参数绑定为函数对象的第二个参数 3. bind1st bind2nd将二元函数对象转为一元函数对 </div> ## 取反适配器 ```C++ #include<iostream> #include<algorithm> #include<functional> #include<vector> using namespace std; //取反适配器 class GreaterThenFive : public unary_function<int,void> { public: bool operator()(int value)const { return value > 5; } }; int main() { vector<int> v; for (int i = 0; i < 10; i++) { v.push_back(i); } vector<int>::iterator it = find_if(v.begin(), v.end(), GreaterThenFive()); if (it != v.end()) { cout << "第一个大于5的数为" << *it << endl; } else { cout << "未找到这样的数"; } //我们在不修改反函数的情况下,需要找到第一个小于5的数 /*取反适配器*/ //1、一元取反 not1 ;二元取反 not2 //2、继承 unary_function<类型1,函数返回值类型> //一元只有一个类型 //3、加const vector<int>::iterator it_fan = find_if(v.begin(), v.end(), not1(GreaterThenFive())); if (it_fan != v.end()) { cout << "第一个小于5的数" << *it_fan << endl;; } else { cout << "未找到这样的数"; } /*我们写一个更高端的写法*/ vector<int>::iterator pos = find_if(v.begin(), v.end(), not1(bind2nd(greater<int>(), 5))); if (pos != v.end()) { cout << "第一个小于5的数" << *pos << endl;; } else { cout << "未找到这样的数"; } return 0; } ``` 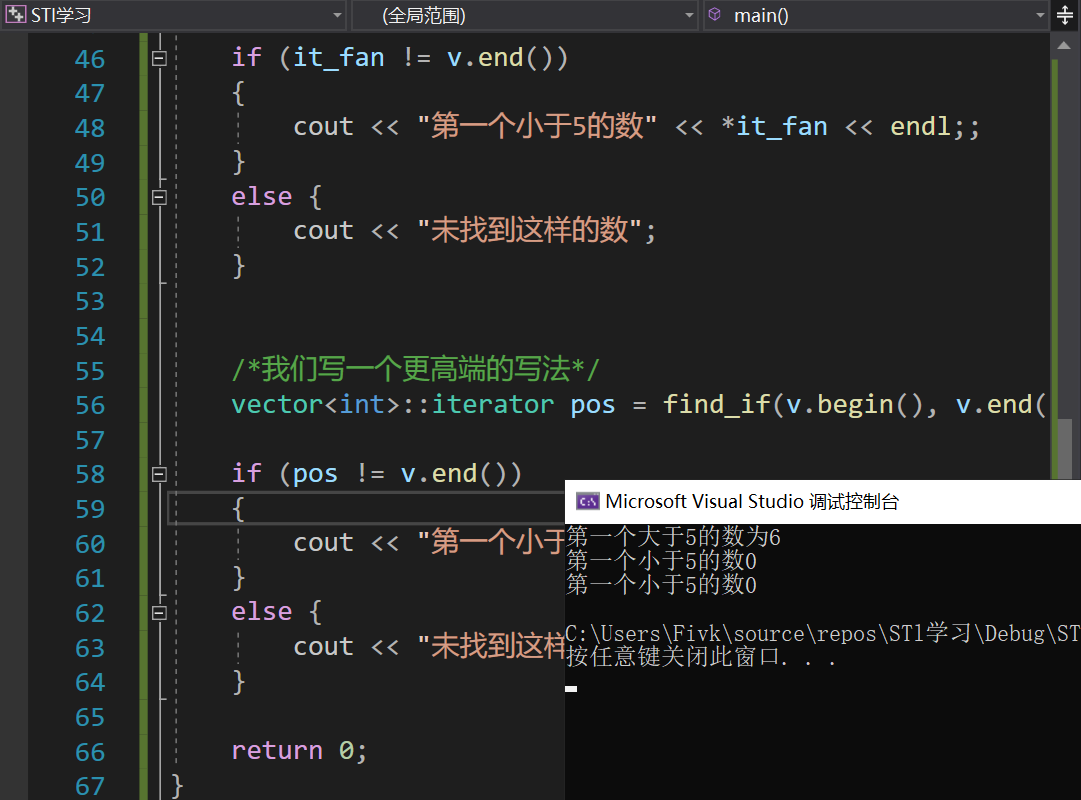 更新ing... 最后修改:2021 年 04 月 20 日 © 禁止转载 打赏 赞赏作者 支付宝微信 赞 如果觉得我的文章对你有用,请随意赞赏
1 条评论
▬▬▬▬.◙.▬▬▬▬
▂▄▄▓▄▄▂
◢◤ █▀▀████▄▄▄▄◢◤
█ 白嫖人员逃跑机 █▀……╬
◥██████◤
══╩══╩═══