Loading... <div class="tip inlineBlock info simple"> Q:稳不稳定? A:稳不稳定我不知道,不过我永远相信`gov.cn`后缀域名网站的稳定性! </div> ## 获取密钥 去天地图注册开发者账号:[http://lbs.tianditu.gov.cn/](http://lbs.tianditu.gov.cn/) 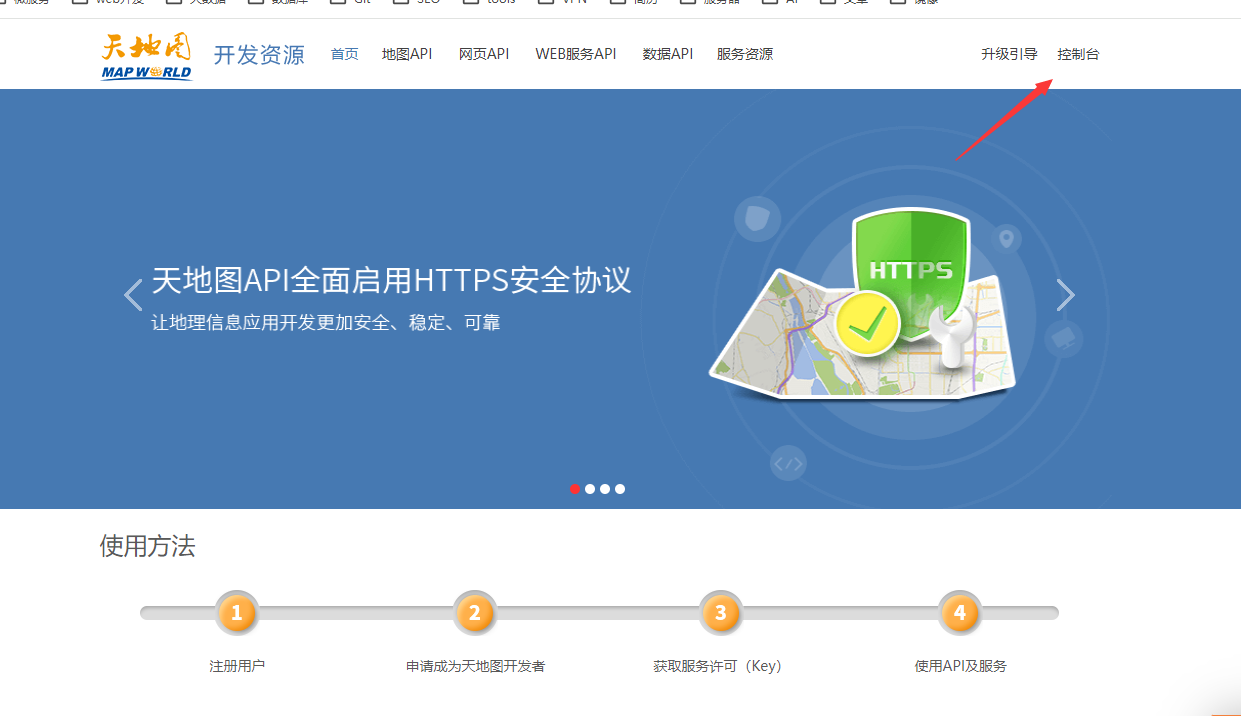 我测试在代码中新建的项目需要选择服务端 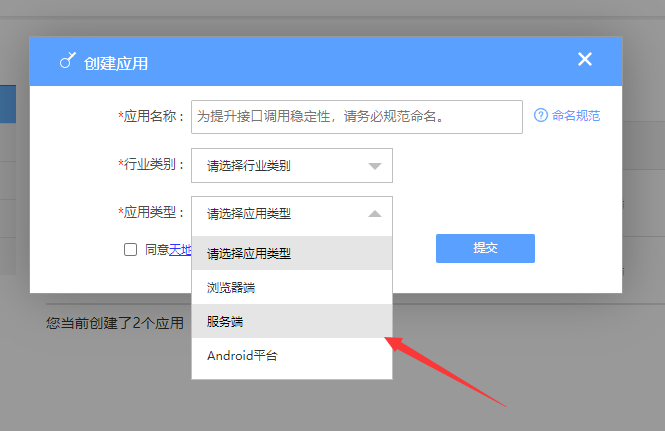 新建之后复制密钥 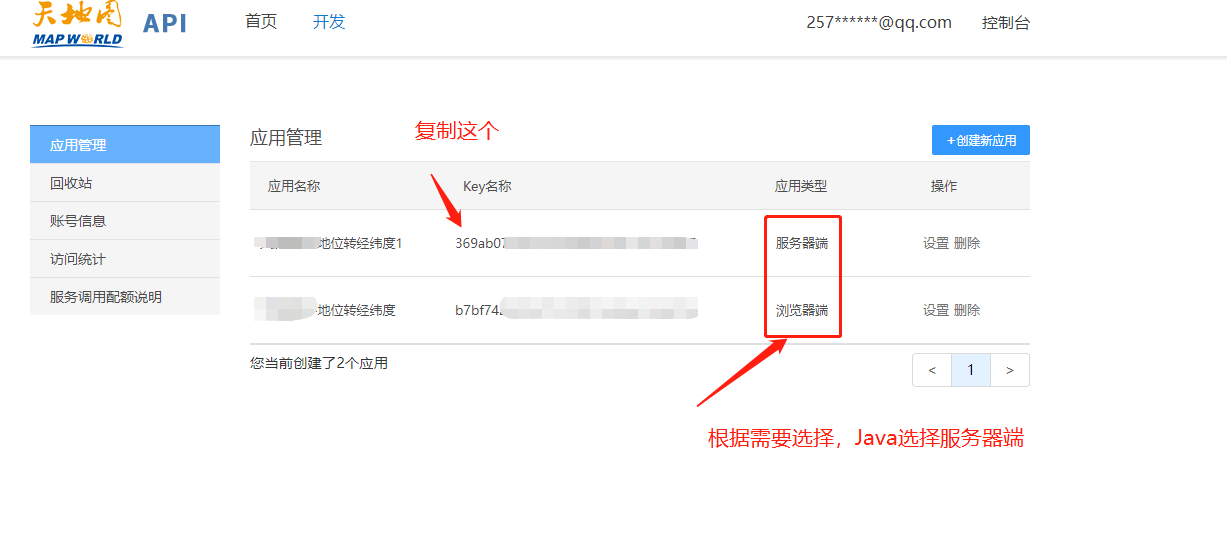 ## 在Java中的代码 首先导入Maven坐标 ```xml <dependency> <groupId>org.snmp4j</groupId> <artifactId>snmp4j</artifactId> <version>2.8.18</version> </dependency> ``` 需要用到的工具类 ```java import lombok.extern.slf4j.Slf4j; import org.apache.http.HttpEntity; import org.apache.http.HttpResponse; import org.apache.http.NameValuePair; import org.apache.http.client.ClientProtocolException; import org.apache.http.client.HttpClient; import org.apache.http.client.config.RequestConfig; import org.apache.http.client.entity.UrlEncodedFormEntity; import org.apache.http.client.methods.HttpDelete; import org.apache.http.client.methods.HttpGet; import org.apache.http.client.methods.HttpPost; import org.apache.http.client.methods.HttpPut; import org.apache.http.client.utils.URLEncodedUtils; import org.apache.http.entity.StringEntity; import org.apache.http.impl.client.HttpClients; import org.apache.http.message.BasicNameValuePair; import java.io.*; import java.net.URI; import java.net.URLEncoder; import java.util.ArrayList; import java.util.List; import java.util.Map; import java.util.Set; @Slf4j public class HttpClientUtil { /** * 封装HTTP POST方法 * * @param * @param * @return * @throws ClientProtocolException * @throws IOException */ public static String post(String url, Map<String, String> paramMap) throws ClientProtocolException, IOException { HttpClient httpClient = HttpClients.createDefault(); HttpPost httpPost = new HttpPost(url); //设置请求和传输超时时间 RequestConfig requestConfig = RequestConfig.custom().setSocketTimeout(60000).setConnectTimeout(60000).build(); httpPost.setConfig(requestConfig); List<NameValuePair> formParams = setHttpParams(paramMap); UrlEncodedFormEntity param = new UrlEncodedFormEntity(formParams, "UTF-8"); httpPost.setEntity(param); HttpResponse response = httpClient.execute(httpPost); log.info("************{}", response); String httpEntityContent = getHttpEntityContent(response); log.info("************{}", httpEntityContent); httpPost.abort(); log.info("************{}", httpEntityContent); return httpEntityContent; } /** * 封装HTTP POST方法 * * @param * @param (如JSON串) * @return * @throws ClientProtocolException * @throws IOException */ public static String post(String url, String data) throws ClientProtocolException, IOException { HttpClient httpClient = HttpClients.createDefault(); HttpPost httpPost = new HttpPost(url); //设置请求和传输超时时间 RequestConfig requestConfig = RequestConfig.custom().setSocketTimeout(60000).setConnectTimeout(60000).build(); httpPost.setConfig(requestConfig); httpPost.setHeader("Content-Type", "text/json; charset=utf-8"); httpPost.setEntity(new StringEntity(URLEncoder.encode(data, "UTF-8"))); HttpResponse response = httpClient.execute(httpPost); String httpEntityContent = getHttpEntityContent(response); httpPost.abort(); return httpEntityContent; } /** * 封装HTTP GET方法 * * @param * @return * @throws ClientProtocolException * @throws IOException */ public static String get(String url) throws ClientProtocolException, IOException { HttpClient httpClient = HttpClients.createDefault(); HttpGet httpGet = new HttpGet(); //设置请求和传输超时时间 RequestConfig requestConfig = RequestConfig.custom().setSocketTimeout(60000).setConnectTimeout(60000).build(); httpGet.setConfig(requestConfig); httpGet.setURI(URI.create(url)); HttpResponse response = httpClient.execute(httpGet); String httpEntityContent = getHttpEntityContent(response); httpGet.abort(); return httpEntityContent; } /** * 封装HTTP GET方法 * * @param * @param * @return * @throws ClientProtocolException * @throws IOException */ public static String get(String url, Map<String, String> paramMap) throws ClientProtocolException, IOException { HttpClient httpClient = HttpClients.createDefault(); HttpGet httpGet = new HttpGet(); //设置请求和传输超时时间 RequestConfig requestConfig = RequestConfig.custom().setSocketTimeout(60000).setConnectTimeout(60000).build(); httpGet.setConfig(requestConfig); List<NameValuePair> formparams = setHttpParams(paramMap); String param = URLEncodedUtils.format(formparams, "UTF-8"); httpGet.setURI(URI.create(url + "?" + param)); HttpResponse response = httpClient.execute(httpGet); String httpEntityContent = getHttpEntityContent(response); httpGet.abort(); return httpEntityContent; } /** * 封装HTTP PUT方法 * * @param * @param * @return * @throws ClientProtocolException * @throws IOException */ public static String put(String url, Map<String, String> paramMap) throws ClientProtocolException, IOException { HttpClient httpClient = HttpClients.createDefault(); HttpPut httpPut = new HttpPut(url); //设置请求和传输超时时间 RequestConfig requestConfig = RequestConfig.custom().setSocketTimeout(60000).setConnectTimeout(60000).build(); httpPut.setConfig(requestConfig); List<NameValuePair> formparams = setHttpParams(paramMap); UrlEncodedFormEntity param = new UrlEncodedFormEntity(formparams, "UTF-8"); httpPut.setEntity(param); HttpResponse response = httpClient.execute(httpPut); String httpEntityContent = getHttpEntityContent(response); httpPut.abort(); return httpEntityContent; } /** * 封装HTTP DELETE方法 * * @param * @return * @throws ClientProtocolException * @throws IOException */ public static String delete(String url) throws ClientProtocolException, IOException { HttpClient httpClient = HttpClients.createDefault(); HttpDelete httpDelete = new HttpDelete(); //设置请求和传输超时时间 RequestConfig requestConfig = RequestConfig.custom().setSocketTimeout(60000).setConnectTimeout(60000).build(); httpDelete.setConfig(requestConfig); httpDelete.setURI(URI.create(url)); HttpResponse response = httpClient.execute(httpDelete); String httpEntityContent = getHttpEntityContent(response); httpDelete.abort(); return httpEntityContent; } /** * 封装HTTP DELETE方法 * * @param * @param * @return * @throws ClientProtocolException * @throws IOException */ public static String delete(String url, Map<String, String> paramMap) throws ClientProtocolException, IOException { HttpClient httpClient = HttpClients.createDefault(); HttpDelete httpDelete = new HttpDelete(); //设置请求和传输超时时间 RequestConfig requestConfig = RequestConfig.custom().setSocketTimeout(60000).setConnectTimeout(60000).build(); httpDelete.setConfig(requestConfig); List<NameValuePair> formparams = setHttpParams(paramMap); String param = URLEncodedUtils.format(formparams, "UTF-8"); httpDelete.setURI(URI.create(url + "?" + param)); HttpResponse response = httpClient.execute(httpDelete); String httpEntityContent = getHttpEntityContent(response); httpDelete.abort(); return httpEntityContent; } /** * 设置请求参数 * * @param * @return */ private static List<NameValuePair> setHttpParams(Map<String, String> paramMap) { List<NameValuePair> formparams = new ArrayList<NameValuePair>(); Set<Map.Entry<String, String>> set = paramMap.entrySet(); for (Map.Entry<String, String> entry : set) { formparams.add(new BasicNameValuePair(entry.getKey(), entry.getValue())); } return formparams; } /** * 获得响应HTTP实体内容 * * @param response * @return * @throws IOException * @throws UnsupportedEncodingException */ private static String getHttpEntityContent(HttpResponse response) throws IOException, UnsupportedEncodingException { HttpEntity entity = response.getEntity(); if (entity != null) { InputStream is = entity.getContent(); BufferedReader br = new BufferedReader(new InputStreamReader(is, "UTF-8")); String line = br.readLine(); StringBuilder sb = new StringBuilder(); while (line != null) { sb.append(line + "\n"); line = br.readLine(); } return sb.toString(); } return ""; } } ``` 主要的代码逻辑 ```java package com.klay.utils; import com.alibaba.fastjson.JSON; import com.alibaba.fastjson.JSONObject; import org.junit.platform.commons.util.StringUtils; import org.slf4j.Logger; import org.slf4j.LoggerFactory; public class AddressUtil { private static final Logger log = LoggerFactory.getLogger(AddressUtil.class); public static String getLatAndLngByAddr(String addr) { try { String result = ""; addr = addr.replace(" ", "").replace("#", "").replace("中国", ""); String queryStr = "http://api.tianditu.gov.cn/geocoder?ds=%7B'keyWord':'" + addr + "'%7D&tk=" + "你获取的key"; String info = com.klay.utils.HttpClientUtil.get(queryStr); if (StringUtils.isNotBlank(info)) { JSONObject resultJson = JSON.parseObject(info); if (!"无结果".equals(resultJson.get("msg"))) { JSONObject locationObj = (JSONObject) resultJson.get("location"); //纬度 String lat = locationObj.get("lat") + ""; //经度 String lng = locationObj.get("lon") + ""; result = "经度:" + lng + "\n纬度:" + lat; } } return result; } catch (Exception e) { return "无"; } } public static void main(String[] args) { System.out.println(getLatAndLngByAddr("福田区梅林一村二期94栋15A室")); } } ``` 最后修改:2024 年 01 月 10 日 © 允许规范转载 打赏 赞赏作者 支付宝微信 赞 如果觉得我的文章对你有用,请随意赞赏