Loading... <div class="tip inlineBlock warning"> 文章为MOOC笔记。 </div> <div class="tip inlineBlock success"> 推荐阅读前先观看MOOC翁凯老师的C语言程序设计进阶 </div> # 先来看一下基本结构 ```c //先了解一下结构 //输入今天日期,输出明天日期 //输入1-7-2021,输出,2021-1-8 #include<stdio.h> #include<stdbool.h> //bool需要 struct date { int month; int day; int year; }; bool isLeap(struct date d); int number0fDays(struct date d); int main(int argc, char const* argv[]) { struct date today, tomorrow; printf("Enter today's date (mm dd yy)"); scanf("%i-%i-%i", &today.month, &today.day, &today.year); if (today.day != number0fDays(today)) { tomorrow.day = today.day + 1; tomorrow.month = today.month; tomorrow.year = today.year; } else if (today.month == 12) { tomorrow.day = 1; tomorrow.month = 1; tomorrow.year = today.year + 1; } else { tomorrow.day = 1; tomorrow.month = today.month + 1; tomorrow.year = today.year; } printf("Tomorrow's date is %i-%i-%i.\n", tomorrow.year, tomorrow.month, tomorrow.day); return 0; } int number0fDays(struct date d) { int daye; const int daysPerMinth[12] = { 31,28,31,30,31,30,31,31,30,31,30,31 }; if (d.month == 2 && isLeap(d)) daye = 29; else daye = daysPerMinth[d.month - 1]; return daye; } bool isLeap(struct date d) { bool leap = false; if ((d.year % 4 == 0 && d.year % 100 != 0) || d.year % 400 == 0) { leap = true; } return leap; } ``` # 如何在结构中传递值? **test1:** ```c #include<stdio.h> struct point { int x; int y; }; void getStruct(struct point); void output(struct point); int main(int argc, char const* argv[]) { struct point y = { 0,0 }; getStruct(y); output(y); } void getStruct(struct point p) { scanf("%d", &p.x); scanf("%d", &p.y); printf("%d,%d\n", p.x, p.y); } void output(struct point p) { printf("%d,%d", p.x, p.y); } ``` * 输入1 2,输出 1 2\n 0 0 * 这个说明了结构函数传递的是结构克隆体,是值,而非数组一样 **test2:** ```c #include<stdio.h> struct point { int x; int y; }; struct point getStruct(void); void output(struct point); int main(int argc, char const* argv[]) { struct point y = { 0,0 }; y = getStruct(); output(y); } struct point getStruct(void) { struct point p; scanf_s("%d", &p.x); scanf_s("%d", &p.y); printf("%d,%d\n", p.x, p.y); return p; } void output(struct point p) { printf("%d,%d", p.x, p.y); } ``` * test2.c带来了结构变量函数直接返回结构即可 # 指向结构的指针 * 前面给出了结构在函数间传递的方法 * 但是对于一个大的结构变量要传进一个函数的话通常更为有效的方法是穿指针,而不是拷贝整个结构 * 因为C语言结构的传递方式是值的传递也就是说在被调用的函数里面要去建立一个和被条用函数一模一样的结构变量,这是一个即费时间又费空间的事情。所以更好的方式是穿指针 <div class="tip inlineBlock info"> 从前面我们知道结构与数组不同,结构名不是地址需要加&取地址 </div> test3: ```c struct date { int month; int day; int year; } myday; struct date* p = &myday; (*p).month = 12; p->month = 12; //上面个两行代码是等价的,通常用第二行 ``` * 用->表示指针所指的结构变量中的成员 test4:改造test2 ```c #include<stdio.h> struct point { int x; int y; }; struct point* getStruct(struct point*); void output(struct point); void print(const struct point* p); int main( int argc, char const* argv[] ) { struct point y = { 0,0 }; getStruct(&y); output(y); print(&y); output(*getStruct(&y)); //这里有一点套娃,认真看 print(getStruct(&y)); } struct point* getStruct(struct point* p) { scanf_s("%d", &p->x); scanf_s("%d", &p->y); printf("%d, %d\n", p->x, p->y); return p; } void output(struct point p) { printf("%d, %d\n", p.x, p.y); } void print(const struct point* p) { printf("%d, %d\n", p->x, p->y); } ``` <div class="tip inlineBlock success"> 所以推荐使用指针传递结构 </div> # 结构中的结构 ## 1.结构数组 **基本样式:** ```c struct date dates[100]; struct date dates[] = { {4,5,2005},{2,4,2005} }; ``` **test5:** ```c #include<stdio.h> struct time { int hour; int minutes; int seconds; }; struct time timeUpdate(struct time now); int main(void) { struct time testTimes[5] = { {11,59,59},{12,0,0},{1,29,59},{23,59,59},{19,12,27} }; int i; for (i = 0; i < 5; ++i) { printf("Time is %.2i:%.2i:%.2i\n\n", testTimes[i].hour, testTimes[i].minutes, testTimes[i].seconds); testTimes[i] = timeUpdate(testTimes[i]); printf("...one second later it's %.2i:%.2i:%.2i\n\n", testTimes[i].hour, testTimes[i].minutes, testTimes[i].seconds); } return 0; } struct time timeUpdate(struct time now) { ++now.seconds; if (now.seconds == 60) { now.seconds = 0; ++now.minutes; if (now.minutes == 60) { now.minutes = 0; ++now.hour; if (now.hour == 24) { now.hour = 0; } } } return now; } ``` 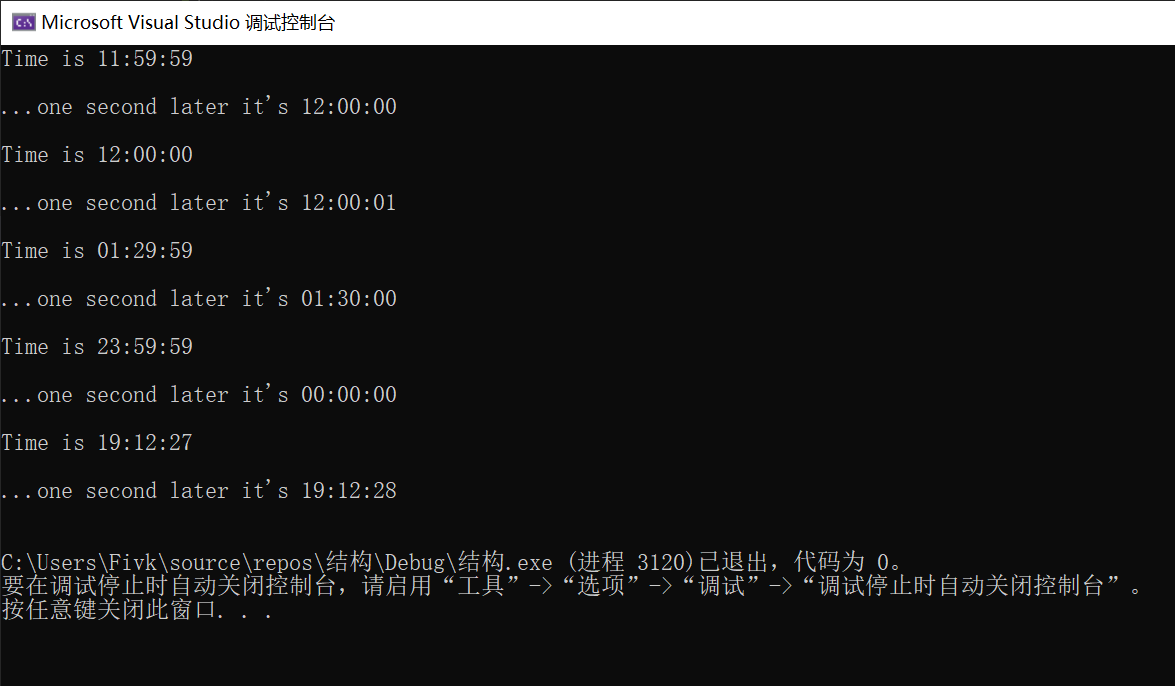 ## 2.结构中的结构 **基本样式:** ```c struct dateAndTime { struct date sdate; struct time stime; }; ``` ###### 嵌套的结构 ```c struct point { int x; int y; }; struct rectangle { struct point pt1; struct point pt2; }; ``` 如果有变量 ```c struct rectangle r; ``` 就可以有: ```c r.pt1.x、r.pt1.y、r.ptl2.x和r.pt2.y ``` 如果有变量定义: ```c struct trctangle r,*rp; rp=&r; ``` 那么下面四种形式是等价的: ```c r.pt1.x rp->pt1.x (r.pt1).x (rp->pt1).x ``` 但是没有以下操作: ```c rp->pt1->x //因为pt1不是指针pr1是结构本身。只有rp是指针 ``` ###### 结构中的结构数组(不解释,但是要看懂第21-24行) ```c #include<stdio.h> struct point { int x; int y; }; struct rectangle { struct point p1; struct point p2; }; void printRect(struct rectangle r) { printf("<%d,%d> to <%d,%d>\n", r.p1.x, r.p1.y, r.p2.y, r.p2.y); } int main(void) { int i; struct rectangle rects[] = { {{1,2},{3,4}}, {{5,6},{7,8} } };//2 rectangles for (i = 0; i < 2; i++) printRect(rects[i]); } ``` **总的来说这不难,只是有点复杂了,层次多了,但并没有超出今天所学的内容。** <div class="tip inlineBlock share"> 我只想说这可以无限套娃,不知不觉0:16了,我还要看秦时明月呢!算了反正习惯了3点睡3点起。 </div> 还有后面习题,1点了。只有两个小时玩的了。 <div class='album_block'> [album type="photos"] [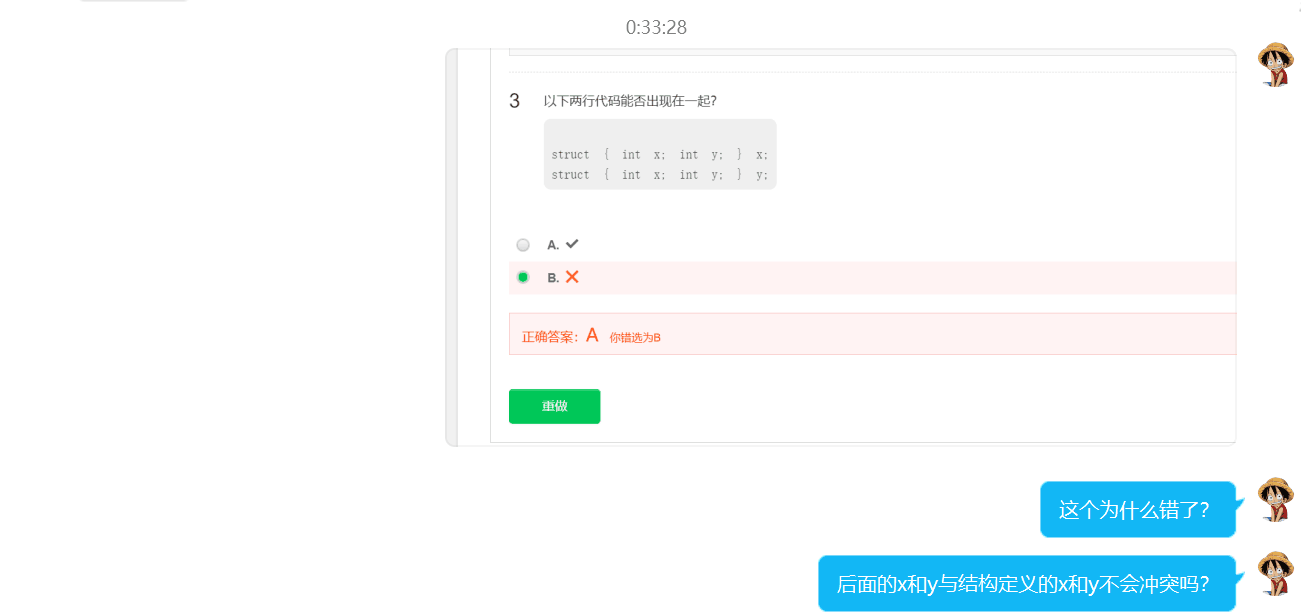 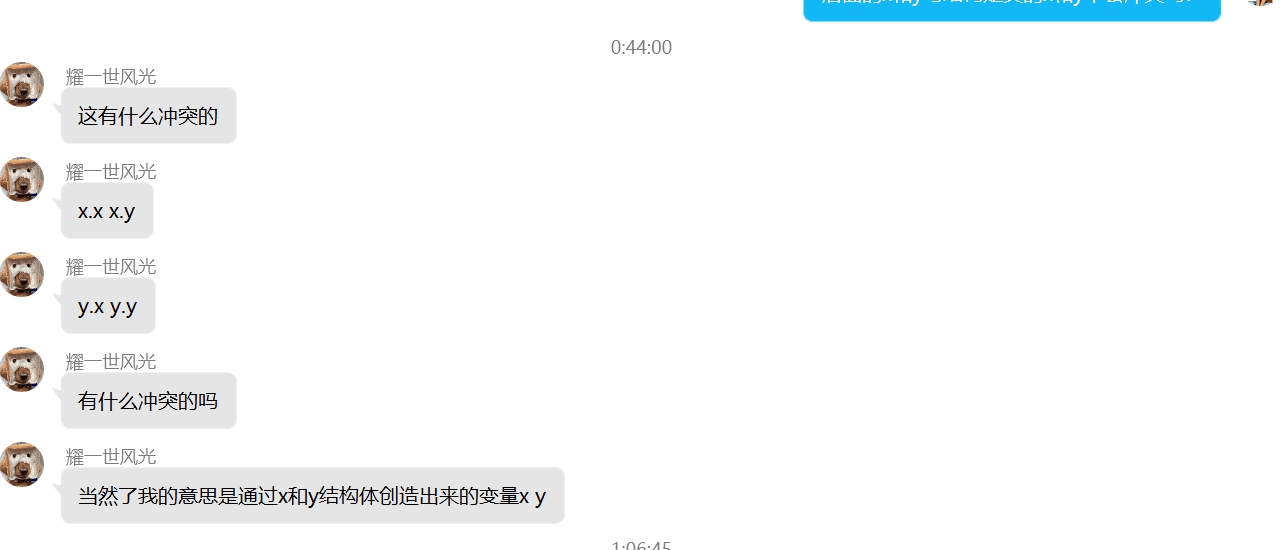 ] [/album] </div> 最后修改:2021 年 02 月 03 日 © 允许规范转载 打赏 赞赏作者 支付宝微信 赞 如果觉得我的文章对你有用,请随意赞赏